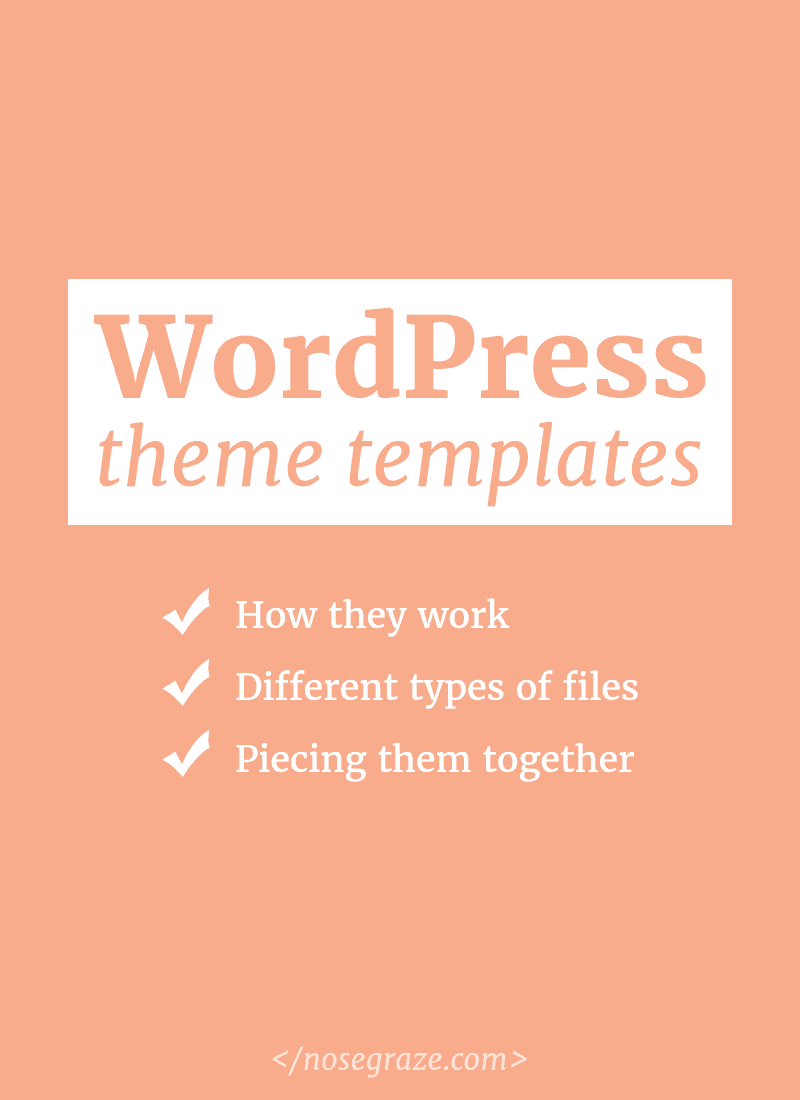
WordPress themes are composed of pieces rather than a single file (as you may see in Blogger).
These pieces are composed of multiple different files that are made up of HTML, CSS, and PHP code. Depending on which page you’re viewing on a WordPress site (homepage, single post, page, archive, etc.), a different file gets called to display that information.
Here’s what a regular “static website” looks like
A static website is just HTML and CSS without the PHP. If you’ve ever taken an HTML class before, you’ve probably made a static website. You put the entire page on a single file, which looks like this:
<!doctype html> <html> <head> <title>My Homepage</title> </head> <body> <header><img src="https://www.nosegraze.com/exampleheader.png" alt="Example site"></header> <nav> <ul> <li><a href="index.html">Homepage</a></li> <li><a href="about.html">About</a></li> <li><a href="contact.html">Contact</a></li> </ul> </nav> <main> <h1>Your layout goes here.</h1> </main> <footer> <p>Copyright © 2016 Ashley Evans.</p> </footer> </body> </html>
Everything is on one page: the header, navigation, main content, and footer. Then when you create a second page, you add all that content again and just change the “main content” area to suit your needs.
What’s the problem with this layout? If you need to edit the logo or the navigation, you have to open up every single page to edit the header bit in each file. What a pain! Imagine having to do this on every single one of your blog posts. There must be a better way, right?
Right!
WordPress uses PHP to avoid repeating code
In order to combat this, WordPress uses some common practices in a scripting language called PHP. With PHP you can include all of your header elements that exist on every page (the <head>
area, logo, navigation) in a separate file called header.php
. Then on all the other pages, you just tell it to include header.php
and it gets included automatically.
The beauty of this is that when you want to edit something in your header, all you have to do is edit one file (header.php
). Then the changes get applied to every single page.
(Of course, that’s not the only reason PHP is used. We also need to use it to pull out information—like posts—from the database.)
The WordPress theme files
So, with the above in mind, WordPress themes aren’t just one single file (like on Blogger). Instead, they’re broken down into multiple files. Some of those files are snippets (like the header, footer, and sidebar area). Others are templates (for posts and pages).
At minimum, here’s a list of files that are usually included in all themes:
- header.php — This is the top bit of your design that needs to be included on every single page. It usually contains your opening
<body>
tag, logo, and navigation bar. - footer.php — This is the bottom of your design. It includes all the footer information that needs to be displayed on every single page. This probably contains the copyright line, footer widgets (if the theme has them), and it lists the JavaScript files. It also contains the closing
</body>
tag. - functions.php — This is sort of a miscellaneous file. It’s 100% PHP and just contains any snippets that need to be used in the theme.
- index.php — This file gets used for your blog homepage. So it deals with displaying a list of your recent posts. However, it’s also the default file for everything else. So if the design doesn’t have a
single.php
file (see below) then theindex.php
is used instead as the default. - single.php — The template for your blog posts. This is the file that gets used when you view an individual post.
- page.php — This is the file for all of your Pages.
- sidebar.php — Includes all the code for your sidebar and including the sidebar widgets.
- style.css — This is where all your CSS and styling lives.
That’s a lot of files! I hope you’re not confused yet. Here’s a more visual breakdown of a typical theme:
This example is for a blog archive that shows a list of blog posts. Imagine that you click on one of those blog posts to be taken to the single post page. The index.php
file would get swapped out for single.php
.
Then imagine that you click over to the “About” page. The single.php
template would be swapped out for page.php
instead.
But the header, footer, and sidebar remain consistent.
Piecing together the files
WordPress has some handy functions you can use for piecing files together. Some of them are:
get_header()
— Include the header.php file.get_footer()
— Include the footer.php file.get_sidebar()
— Include the sidebar.php file.
So a sample index.php
file might look like this:
<?php get_header(); ?> <main id="main" class="site-main" role="main"> <!-- Blog posts would go here --> </main> <?php get_sidebar(); get_footer();
This helps keep the various files clean, to the point, and avoid repeating the same HTML in different places!
Hi Ashley. I have been a reader of your blog for a few months and really like your clear simple tech explanation. This one really removed the fear that I have of PHP, so thanks a lot!
You’re so welcome! 🙂
Maybe someday I’ll be able to put it all together! LOL